
These are the ‘First’, ‘Second’, and ‘Third’ strings. We create a new Queue, then add some items to it. Once again we’ll test this out in the interactive Python interpreter.

If you try to pop on an empty queue, you’ll get an error. Before you go trying to take items out of the queue, however, you should make sure there are some items to process from the queue. Since this is the case, we can use the list’s built-in pop() method, and we’ll always get back the front-most item of the queue because the pop method always returns the last item in the list. The front of the queue is actually the end of the list. Now let’s create the dequeue() method which will help us get items out of the queue. If this was a line at the store, the items on the right will be processed first. Now we can check the contents of the items, and we can see that they are in the correct order. Finally, we add the string ‘Last in line’. Then we add another string ‘Second in line’. This would be the first item to be processed from the queue. First, we put the string ‘Got in line first’ into the queue. We create a Queue object first and then enqueue an item into it. We can go back to the terminal to test this out in the interactive Python shell.
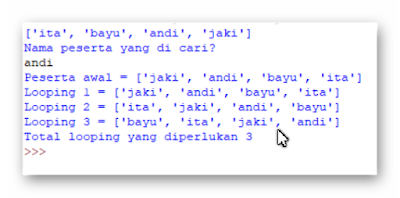
The reason we do that is that we want to save the end of the list for popping items off and use the front of the list for inserting. We want to insert the item into the zeroth index of the list, or in other words the very first position of the list. With a queue, we will not be appending the item to the end of the list, like is done with a stack. Inside the body of our method, we’re want to insert this item into the list in a queue-like fashion. For this method, we have to pass in as a parameter the item that we want to add into the queue.
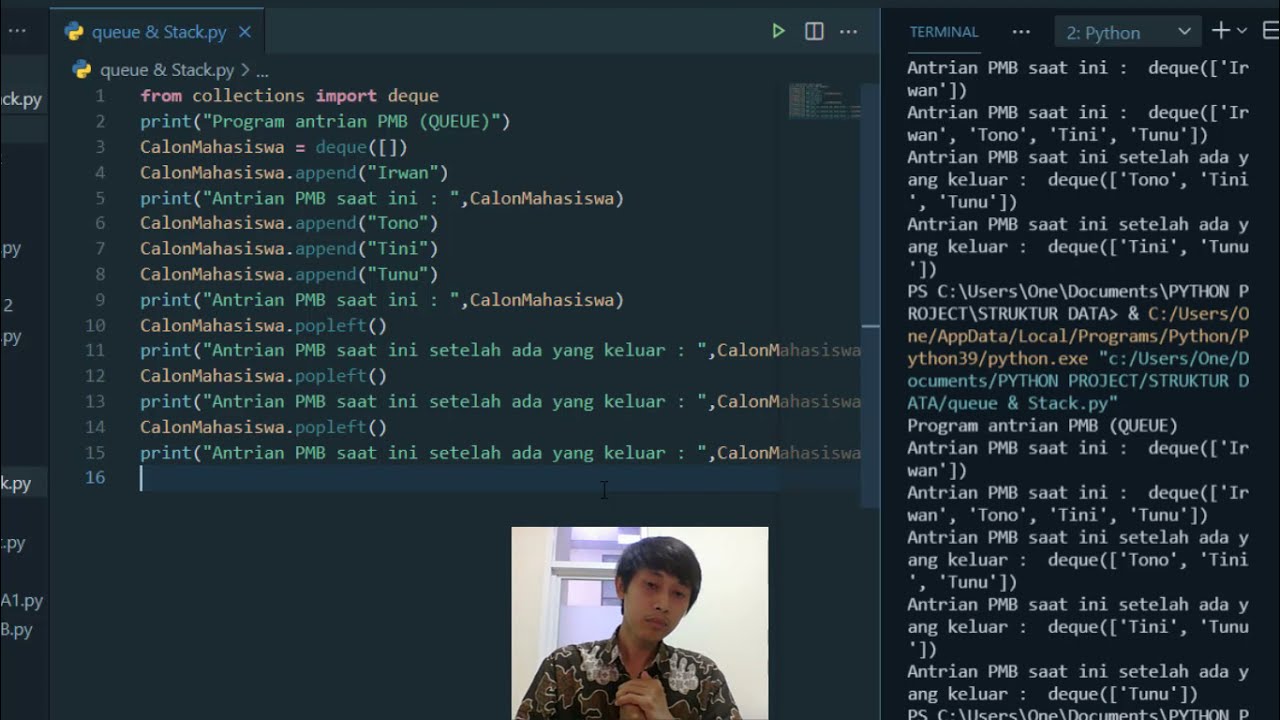
We can now start coding the methods we need for our Queue class. We also want to be able to check for the size of the queue using size(), and whether the queue is empty using is_empty(). To check on want the next item in the queue that’s going to be removed we can use a peek() method. The dequeue() method doesn’t need an item parameter because we’re always going to be popping off the end of the list which automatically takes the last item of the list for us. The enqueue() method needs a parameter of item so that we can add it to the queue. To set up the basic functionality needed to get things into and out of our queue, we can stub out an enqueue() for adding and a dequeue() for removing. In the _init_ method, we can initialize items to an empty list. The class will simply be named Queue, and we’ll represent our queue as a list. A Queue Classįor this example of a queue in Python, we’ll use a class that will have all the methods needed to implement the functions of a queue. Now let’s look at examples of a queue in Python. In addition, queues also preserve order so when you think about people joining a line, the order is preserved there as well. This is different from stacks which are last-in-first-out. This is called first-in-first-out or FIFO.
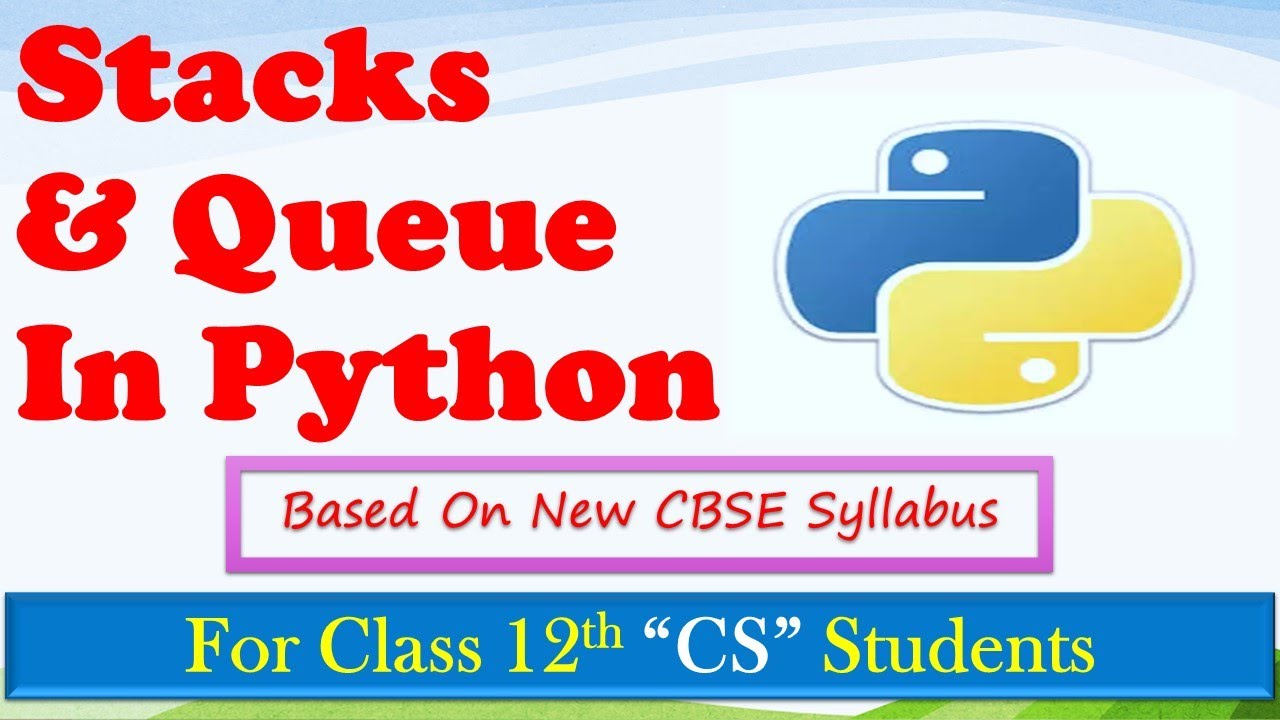
If you think of a queue as a line of customers, a customer adds themselves to the end of the line and eventually leaves from the front of the line. The last time you went grocery shopping you likely had to wait in a line to check out. Items that are added to the back of a queue and removed from the front of the queue. Queues hold a collection of items in the order in which they were added. Queues are a linear abstract data type with some key differences between stacks.
